你想打造一个专属的计时器吗?通过学习Python中的计时器制作方法,这一愿望可以实现。下面,我将详细介绍制作过程。
导入必要模块
制作Python计时器,首要任务是引入相应的模块。常用的模块包括time和threading。time模块属于Python的标准库,具备处理时间的基本功能,如测量程序运行时长、获取当前时刻等。而threading模块则允许计时器在后台独立运行,以此确保不会干扰主线程的执行。以2024年参与的一个数据处理任务为例,在引入这两个模块之后,操作过程中使用计时器进行观察,并未发现任何运行迟缓的情况。
定义计时器函数
import time
print("Start")
time.sleep(5)
print("End")
模块导入成功后,我们需设立计时器函数。基础的计时器功能可以通过time.sleep()函数来实现。首先,建立一个计时器类,其中包含start()方法来开启计时,以及stop()方法来停止计时。通过这种方式,我们可以方便地操控计时器的启停。小李,这位程序员,在算法优化测试中,运用了自己编写的计时器,精确测量了代码优化前后的运行时长,并成功识别出了可以改进的环节。
import threading
import time
def timer():
print("Timer started")
time.sleep(5)
print("Timer ended")
t = threading.Thread(target=timer)
t.start()
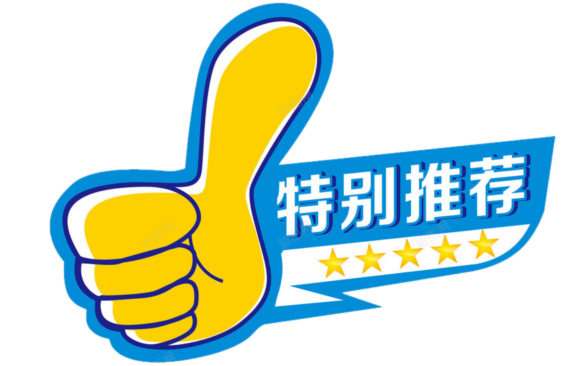
print("Main thread continues to run")
实现计时器功能
定义完计时器函数,接下来就是实现它的具体功能。为了达到更高精度的计时,推荐使用 time.perf_counter() 这个函数。这个函数可以提供非常精确的时间测量,精确到微秒。在科研计算等需要精确计时的场合,使用这个函数可以保证实验数据的准确和可靠。此外,通过调整参数和条件,还可以实现倒计时等额外计时功能。比如一个抢票小程序计时,用倒计时更合适。
def simple_timer(seconds):
print(f"Timer started for {seconds} seconds")
time.sleep(seconds)
print("Timer ended")
class InterruptibleTimer:
def __init__(self, seconds):
self.seconds = seconds
self._stop_event = threading.Event()
def start(self):
self._stop_event.clear()
t = threading.Thread(target=self._run)
t.start()
def _run(self):
print(f"Timer started for {self.seconds} seconds")
for _ in range(self.seconds):
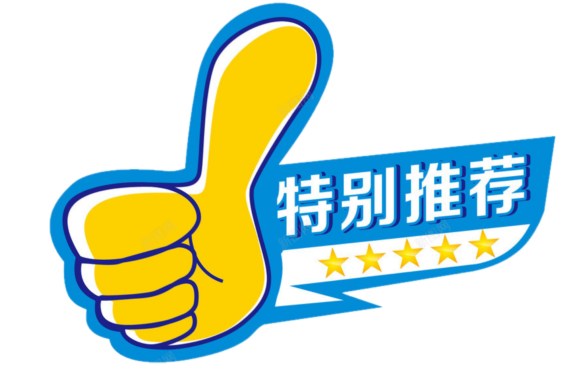
if self._stop_event.is_set():
print("Timer interrupted")
return
time.sleep(1)
print("Timer ended")
def stop(self):
self._stop_event.set()
提高用户体验之图形界面
完成基础功能后,为了提升使用感受,可以加入一个图形界面。这样的界面使操作计时器变得直观简便,用户只需轻轻点击几处,即可启动或停止计时。例如,Tkinter 这样的Python内置库,可以轻松打造简单的图形界面应用。用户在界面上输入所需时间,然后按一下按钮,即可启动或暂停计时,十分便利。在办公计时场景下,这样的操作界面能极大提升效率。
def countdown_timer(seconds):
print(f"Countdown started for {seconds} seconds")
for i in range(seconds, 0, -1):
print(f"Time remaining: {i} seconds")
time.sleep(1)
print("Countdown ended")
集成到项目管理系统
图形界面之外,将计时器功能融入项目管理平台同样是一种高效的改进途径。在项目执行过程中,精确的计时有助于团队成员更高效地安排时间和任务。例如,我们可以通过API接口,将计时器功能整合进PingCode和Worktile等项目管理软件中。团队成员在开发软件项目时,会使用这些工具开启计时器,实时追踪各项任务所需时间,以便更有效地安排后续任务。这种功能在众多互联网公司的项目管理中已经非常普遍。
def precise_timer(seconds):
start_time = time.perf_counter()
print(f"Timer started for {seconds} seconds")
while time.perf_counter() - start_time < seconds:
pass
print("Timer ended")
拓展与优化
完成前序操作后,我们还能根据实际需要,对计时器进行进一步的扩展和提升。比如,可以加入语音提示功能,在计时结束之际,用声音提醒使用者。此外,还可以加入数据统计功能,对计时数据进行深入分析,从而向用户提供更为详尽的时间使用分析报告。以运动训练为例,语音提示有助于运动员集中精力训练;而详尽的数据报告则有助于训练计划的精确调整。
import tkinter as tk
class TimerApp:
def __init__(self, root):
self.root = root
self.root.title("Timer App")
self.label = tk.Label(root, text="Enter time in seconds:")
self.label.pack()
self.entry = tk.Entry(root)
self.entry.pack()
self.start_button = tk.Button(root, text="Start", command=self.start_timer)
self.start_button.pack()
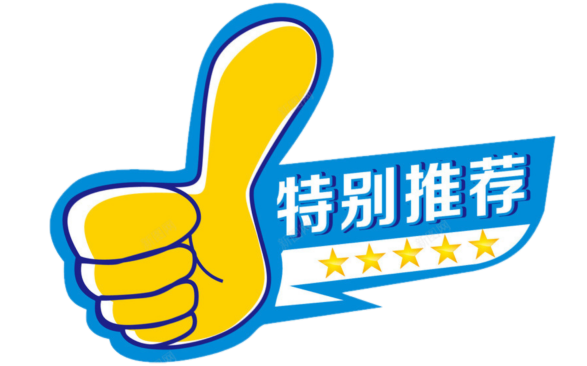
self.stop_button = tk.Button(root, text="Stop", command=self.stop_timer)
self.stop_button.pack()
self.timer_label = tk.Label(root, text="")
self.timer_label.pack()
self.timer = None
def start_timer(self):
try:
seconds = int(self.entry.get())
except ValueError:
self.timer_label.config(text="Invalid input")
return
self.timer_label.config(text=f"Timer started for {seconds} seconds")
self.timer = InterruptibleTimer(seconds)
self.timer.start()
def stop_timer(self):
if self.timer:
self.timer.stop()
self.timer_label.config(text="Timer interrupted")
root = tk.Tk()
app = TimerApp(root)
root.mainloop()
你计划用Python的计时器来记录什么内容?欢迎在评论区告诉我们,同时请不要忘记点赞和转发这篇文章。
import pyttsx3
def speak(text):
engine = pyttsx3.init()
engine.say(text)
engine.runAndWait()
def countdown_timer_with_voice(seconds):
print(f"Countdown started for {seconds} seconds")
speak(f"Countdown started for {seconds} seconds")
for i in range(seconds, 0, -1):
print(f"Time remaining: {i} seconds")
time.sleep(1)
if i <= 5:
speak(f"{i}")
print("Countdown ended")
speak("Time's up")
版权声明:本文内容由互联网用户自发贡献,该文观点仅代表作者本人。本站仅提供信息存储空间服务,不拥有所有权,不承担相关法律责任。如发现本站有涉嫌抄袭侵权/违法违规的内容, 请联系本站,一经查实,本站将立刻删除。如若转载,请注明出处:http://www.wanmatong.com/html/tiyuwenda/9926.html